Creating an HTML5 slot machine can be a fun and rewarding project for web developers. This tutorial will guide you through the process of building a simple slot machine using HTML5, CSS, and JavaScript. By the end of this tutorial, you’ll have a fully functional slot machine that you can customize and expand upon. Prerequisites Before you start, make sure you have a basic understanding of the following: HTML5 CSS3 JavaScript Step 1: Setting Up the HTML Structure First, let’s create the basic HTML structure for our slot machine.
Beste casinoer india 2024
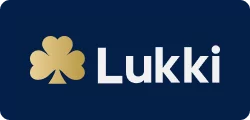
- 24/7 live chat
- Spesielt VIP-program
- Celestial Bet
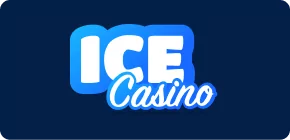
- Regular promotions
- Deposit with Visa
- Luck&Luxury
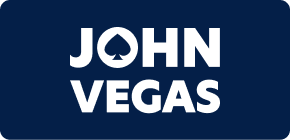
- Regular promotions
- Deposit with Visa
- Royal Wins
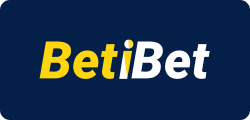
- Regular promotions
- Deposit with Visa
- Win Big Now
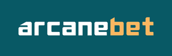
- Regular promotions
- Deposit with Visa
- Luxury Play
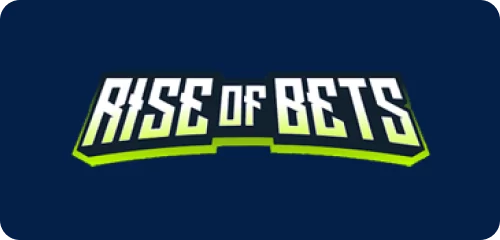
- Regular promotions
- Deposit with Visa
- Elegance+Fun
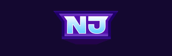
- Regular promotions
- Deposit with Visa
- Opulence & Fun
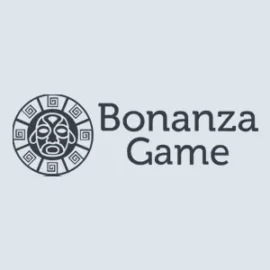
- Regular promotions
- Deposit with Visa
- Luck&Luxury
- html5 slot machine tutorial
- free html5 slot machine source code
- free html5 slot machine source code
- giochi slot machine gratis senza soldi
- About html5 slot machine tutorial FAQ
html5 slot machine tutorial
Creating an HTML5 slot machine can be a fun and rewarding project for web developers. This tutorial will guide you through the process of building a simple slot machine using HTML5, CSS, and JavaScript. By the end of this tutorial, you’ll have a fully functional slot machine that you can customize and expand upon.
Prerequisites
Before you start, make sure you have a basic understanding of the following:
- HTML5
- CSS3
- JavaScript
Step 1: Setting Up the HTML Structure
First, let’s create the basic HTML structure for our slot machine.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML5 Slot Machine</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="slot-machine">
<div class="reels">
<div class="reel"></div>
<div class="reel"></div>
<div class="reel"></div>
</div>
<button class="spin-button">Spin</button>
</div>
<script src="script.js"></script>
</body>
</html>
Explanation:
<div class="slot-machine">
: This container holds the entire slot machine.<div class="reels">
: This container holds the individual reels.<div class="reel">
: Each reel will display a symbol.<button class="spin-button">
: This button will trigger the spin action.
Step 2: Styling the Slot Machine with CSS
Next, let’s add some CSS to style our slot machine.
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f0f0f0;
font-family: Arial, sans-serif;
}
.slot-machine {
background-color: #333;
padding: 20px;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.5);
}
.reels {
display: flex;
justify-content: space-between;
margin-bottom: 20px;
}
.reel {
width: 100px;
height: 100px;
background-color: #fff;
border: 2px solid #000;
display: flex;
justify-content: center;
align-items: center;
font-size: 24px;
font-weight: bold;
}
.spin-button {
width: 100%;
padding: 10px;
font-size: 18px;
cursor: pointer;
}
Explanation:
body
: Centers the slot machine on the page..slot-machine
: Styles the main container of the slot machine..reels
: Arranges the reels in a row..reel
: Styles each individual reel..spin-button
: Styles the spin button.
Step 3: Adding Functionality with JavaScript
Now, let’s add the JavaScript to make the slot machine functional.
const reels = document.querySelectorAll('.reel');
const spinButton = document.querySelector('.spin-button');
const symbols = ['🍒', '🍋', '🍇', '🔔', '⭐', '💎'];
function getRandomSymbol() {
return symbols[Math.floor(Math.random() * symbols.length)];
}
function spinReels() {
reels.forEach(reel => {
reel.textContent = getRandomSymbol();
});
}
spinButton.addEventListener('click', spinReels);
Explanation:
reels
: Selects all the reel elements.spinButton
: Selects the spin button.symbols
: An array of symbols to be displayed on the reels.getRandomSymbol()
: A function that returns a random symbol from thesymbols
array.spinReels()
: A function that sets a random symbol for each reel.spinButton.addEventListener('click', spinReels)
: Adds an event listener to the spin button that triggers thespinReels
function when clicked.
Step 4: Testing and Customization
Open your HTML file in a browser to see your slot machine in action. Click the “Spin” button to see the reels change.
Customization Ideas:
- Add More Reels: You can add more reels by duplicating the
.reel
divs inside the.reels
container. - Change Symbols: Modify the
symbols
array to include different icons or text. - Add Sound Effects: Use the Web Audio API to add sound effects when the reels spin or when a winning combination is achieved.
- Implement a Win Condition: Add logic to check for winning combinations and display a message when the player wins.
Congratulations! You’ve built a basic HTML5 slot machine. This project is a great way to practice your web development skills and can be expanded with additional features like animations, sound effects, and more complex game logic. Happy coding!
free html5 slot machine source code
Creating an HTML5 slot machine can be a fun and rewarding project, especially if you’re looking to dive into web development or game design. The good news is that there are plenty of free resources available to help you get started. In this article, we’ll explore where you can find free HTML5 slot machine source code and how you can use it to build your own game.
Benefits of Using Free HTML5 Slot Machine Source Code
Before diving into the resources, let’s discuss why using free source code can be beneficial:
- Cost-Effective: Free source code eliminates the need for expensive licenses or subscriptions.
- Time-Saving: You can skip the initial development phase and focus on customization and optimization.
- Learning Opportunity: Studying existing code can help you understand best practices and improve your coding skills.
Where to Find Free HTML5 Slot Machine Source Code
1. GitHub
GitHub is a treasure trove for developers, and you can find a plethora of free HTML5 slot machine source code repositories. Here are a few to get you started:
- Slot Machine Game: A GitHub topic that aggregates various slot machine game repositories.
- HTML5 Slot Machine: Another GitHub topic specifically for HTML5 slot machines.
2. OpenGameArt.org
OpenGameArt.org is a community-driven site that offers free game assets, including source code. While you may need to search a bit, you can often find complete game projects, including slot machines.
- Slot Machine: Search for slot machine-related assets and projects.
3. CodePen
CodePen is a social development environment where developers share their work. You can find HTML5 slot machine demos and source code snippets that you can adapt for your project.
- Slot Machine: Search for slot machine-related pens.
4. FreeCodeCamp
FreeCodeCamp offers a variety of free coding resources, including tutorials and projects. Sometimes, these projects include source code for HTML5 games, including slot machines.
- HTML5 Game Development: Look for game development tutorials that include slot machine projects.
How to Use Free HTML5 Slot Machine Source Code
Once you’ve found a suitable source code, here’s how you can use it:
1. Download the Source Code
- GitHub: Clone the repository using Git or download it as a ZIP file.
- OpenGameArt.org: Download the project files directly.
- CodePen: Fork the pen to your own account or download the HTML, CSS, and JavaScript files.
- FreeCodeCamp: Follow the tutorial to download or clone the project.
2. Set Up Your Development Environment
- Text Editor: Use a text editor like Visual Studio Code, Sublime Text, or Atom.
- Local Server: Set up a local server using tools like XAMPP, WAMP, or Node.js.
3. Customize the Code
- Graphics and Assets: Replace the default graphics and assets with your own designs.
- Logic and Mechanics: Modify the game logic and mechanics to suit your vision.
- Responsive Design: Ensure the slot machine is responsive and works well on different devices.
4. Test and Debug
- Browser Testing: Test the slot machine in various browsers (Chrome, Firefox, Safari, etc.).
- Debugging Tools: Use browser developer tools to debug any issues.
5. Deploy Your Slot Machine
- Hosting: Choose a hosting provider like GitHub Pages, Netlify, or Firebase.
- Domain: Optionally, purchase a custom domain name.
- SEO and Analytics: Implement SEO best practices and set up analytics to track user engagement.
Creating an HTML5 slot machine doesn’t have to be a daunting task, especially with the wealth of free source code available. By leveraging these resources, you can build a fully functional and customizable slot machine game. Whether you’re a beginner or an experienced developer, these tools and tutorials will help you bring your vision to life. Happy coding!

free html5 slot machine source code
Creating an HTML5 slot machine can be a fun and educational project, whether you’re a developer looking to enhance your skills or a business owner aiming to offer an engaging online game. Fortunately, there are several free resources available that provide HTML5 slot machine source code. This article will guide you through the process of finding and utilizing these resources effectively.
Benefits of Using Free HTML5 Slot Machine Source Code
- Cost-Effective: Free source code eliminates the need for expensive development costs.
- Time-Saving: Pre-built code can significantly reduce development time.
- Customization: Most free source codes are open-source, allowing for easy customization.
- Learning Opportunity: Great for developers looking to understand game mechanics and HTML5 technologies.
Where to Find Free HTML5 Slot Machine Source Code
1. GitHub
GitHub is a treasure trove for developers, hosting numerous open-source projects. Here are some repositories you might find useful:
- Slot Machine Game: A simple, well-documented slot machine game.
- HTML5 Slot Machine: A more advanced project with multiple features.
2. CodePen
CodePen is a social development environment where you can find and share code snippets. Here are some pens that might interest you:
- HTML5 Slot Machine: A basic slot machine with a clean interface.
- Responsive Slot Machine: A responsive design suitable for mobile devices.
3. OpenGameArt
OpenGameArt is a community-driven site offering free game assets, including source code. Here are some resources:
- Slot Machine Source Code: A comprehensive package with assets and code.
How to Use the Source Code
1. Download the Source Code
- Navigate to the repository or pen.
- Click on the “Download” or “Fork” button to get a copy of the code.
2. Set Up Your Development Environment
- Install a code editor like Visual Studio Code.
- Set up a local server if required (e.g., using XAMPP or Node.js).
3. Customize the Code
- Open the downloaded files in your code editor.
- Modify the HTML, CSS, and JavaScript files to suit your needs.
- Add your own graphics, sounds, and animations.
4. Test the Slot Machine
- Run the game on your local server.
- Test for functionality, responsiveness, and compatibility across different browsers and devices.
5. Deploy the Game
- Upload the files to your web server.
- Ensure all assets are correctly linked.
- Test the live version to ensure everything works as expected.
Tips for Customization
- Graphics: Replace default images with your own designs to create a unique look.
- Sound Effects: Add custom sound effects to enhance the gaming experience.
- Game Logic: Modify the JavaScript to change the game’s rules, such as win conditions and payout rates.
- Responsive Design: Ensure the game is fully responsive to provide a seamless experience on all devices.
Free HTML5 slot machine source code offers a fantastic opportunity to create engaging games without significant financial investment. By leveraging platforms like GitHub, CodePen, and OpenGameArt, you can find high-quality resources to kickstart your project. Remember to customize the code to make it your own and thoroughly test your game before deployment. Happy coding!
giochi slot machine gratis senza soldi
Introduzione
I giochi slot machine gratis senza soldi sono diventati una delle opzioni più popolari per gli appassionati di gioco d’azzardo. Questi giochi offrono l’opportunità di divertirsi e di testare nuove strategie senza rischiare denaro reale. In questo articolo, esploreremo tutto ciò che c’è da sapere sui giochi slot machine gratis, dalle loro caratteristiche principali ai migliori siti per giocarci.
Vantaggi dei Giochi Slot Machine Gratis
1. Nessun Rischio Finanziario
- Senza Deposito: Non è necessario depositare denaro reale per giocare.
- Senza Perdite: Non si rischia di perdere denaro, il che rende il gioco più rilassante.
2. Testare Nuove Strategie
- Esperimenti: Puoi provare diverse strategie e tattiche senza preoccuparti delle conseguenze finanziarie.
- Apprendimento: Migliora le tue abilità e comprendi meglio il meccanismo dei giochi.
3. Accesso a Nuovi Giochi
- Varietà: I siti offrono una vasta gamma di slot machine gratis, permettendoti di esplorare nuovi giochi.
- Innovazione: Rimani aggiornato sulle ultime tendenze e innovazioni nel mondo dei giochi d’azzardo.
Come Trovare i Migliori Siti per Giochi Slot Machine Gratis
1. Ricerche Online
- Recensioni: Leggi recensioni e feedback di altri giocatori per trovare i migliori siti.
- Forum: Partecipa a forum di gioco d’azzardo per ottenere consigli e raccomandazioni.
2. Caratteristiche Chiave
- Selezione di Giochi: Assicurati che il sito offra una vasta selezione di slot machine gratis.
- Interfaccia Utente: Una buona interfaccia utente rende il gioco più piacevole e facile da navigare.
- Sicurezza: Verifica che il sito sia sicuro e affidabile per proteggere i tuoi dati personali.
3. Bonus e Promozioni
- Bonus di Benvenuto: Alcuni siti offrono bonus di benvenuto per i nuovi giocatori.
- Promozioni Periodiche: Cerca siti che offrono promozioni e tornei regolari.
Consigli per Massimizzare l’Esperienza di Gioco
1. Imposta un Budget
- Tempo di Gioco: Stabilisci un limite di tempo per il tuo gioco per evitare di perdere il controllo.
- Fiducia in Se Stessi: Gioca in modo responsabile e non lasciarti influenzare dai risultati.
2. Sfrutta i Tutorial
- Guide: Utilizza guide e tutorial disponibili online per imparare le regole e le strategie dei giochi.
- Video: Guarda video di giocatori esperti per ottenere suggerimenti pratici.
3. Partecipa a Comunità di Gioco
- Social Media: Segui pagine e gruppi di social media dedicati ai giochi d’azzardo per rimanere aggiornato.
- Chat Online: Partecipa a chat online per condividere esperienze e consigli con altri giocatori.
Conclusione
I giochi slot machine gratis senza soldi offrono un’ottima opportunità per divertirsi, testare nuove strategie e esplorare una vasta gamma di giochi senza rischi finanziari. Seguendo i consigli e le raccomandazioni forniti in questo articolo, puoi massimizzare la tua esperienza di gioco e goderti al meglio questa forma di intrattenimento. Ricorda sempre di giocare in modo responsabile e di non lasciarti influenzare dai risultati.

About html5 slot machine tutorial FAQ
🤔 How to Create a Slot Machine Using HTML5: A Step-by-Step Tutorial?
Creating a slot machine using HTML5 involves several steps. First, design the layout with HTML, including reels and buttons. Use CSS for styling, ensuring a visually appealing interface. Next, implement JavaScript to handle the slot machine's logic, such as spinning the reels and determining outcomes. Use event listeners to trigger spins and update the display. Finally, test thoroughly for responsiveness and functionality across different devices. This tutorial provides a foundational understanding, enabling you to create an interactive and engaging slot machine game.
🤔 What are the best sources for free HTML5 slot machine source code?
Discovering free HTML5 slot machine source code can be a game-changer for developers. Top sources include GitHub, where numerous open-source projects offer customizable code. Websites like CodePen and JSFiddle showcase user-created HTML5 games, including slot machines, often with editable code snippets. Additionally, specialized forums such as Stack Overflow and Reddit's r/gamedev can provide valuable insights and links to free resources. For a more curated experience, platforms like FreeHTML5.co offer free HTML5 templates, some of which include slot machine games. Always ensure to check the licensing terms to avoid any legal issues.
🤔 What is the Best Way to Build a Slot Machine with HTML5 and JavaScript?
Building a slot machine with HTML5 and JavaScript involves creating a visually appealing interface using HTML5 for structure and CSS for styling, and implementing the game logic with JavaScript. Start by designing the reels and symbols using HTML5 elements like . Use CSS to animate the spinning effect. In JavaScript, handle user interactions, such as button clicks to start and stop the reels. Implement random symbol generation and check for winning combinations. Ensure the game is responsive and mobile-friendly. Test thoroughly across different browsers and devices to ensure compatibility and smooth performance. This approach leverages the power of modern web technologies to create an engaging and interactive slot machine experience.
🤔 What are the best practices for designing a slot machine in HTML?
Designing a slot machine in HTML involves several best practices. First, use semantic HTML elements like
🤔 What are the top-rated slot machine casino games available on CodeCanyon?
CodeCanyon offers a variety of top-rated slot machine casino games that are highly customizable and feature-rich. Some of the best options include 'Slot Machine Game - HTML5 Casino Game,' known for its stunning graphics and smooth gameplay. Another popular choice is 'Multi Slot - HTML5 Casino Slot Game,' which supports multiple themes and offers extensive customization options. For those seeking a more classic experience, 'Classic Slot Machine - HTML5 Casino Game' provides a nostalgic feel with modern features. These games are designed to engage players and can be easily integrated into any casino platform, making them ideal for developers and operators alike.
🤔 What are the best practices for designing a slot machine in HTML?
Designing a slot machine in HTML involves several best practices. First, use semantic HTML elements like
🤔 How can I play the free OMG Puppies slot machine game?
To play the free OMG Puppies slot machine game, visit an online casino that offers free-to-play versions of slot games. Look for a reputable site that provides demo versions of popular slots. Once on the site, search for OMG Puppies or browse through the slot categories. Click on the game to load it, and you'll be able to play without needing to deposit any money. Ensure your browser supports Flash or HTML5 for seamless gameplay. Enjoy the adorable puppies and exciting features of OMG Puppies for free!
🤔 What are the best sources for free HTML5 slot machine source code?
Discovering free HTML5 slot machine source code can be a game-changer for developers. Top sources include GitHub, where numerous open-source projects offer customizable code. Websites like CodePen and JSFiddle showcase user-created HTML5 games, including slot machines, often with editable code snippets. Additionally, specialized forums such as Stack Overflow and Reddit's r/gamedev can provide valuable insights and links to free resources. For a more curated experience, platforms like FreeHTML5.co offer free HTML5 templates, some of which include slot machine games. Always ensure to check the licensing terms to avoid any legal issues.
🤔 Where can I find free HTML5 slot machine source code?
You can find free HTML5 slot machine source code on various online platforms. GitHub offers numerous repositories with open-source projects, including slot machines. Websites like CodePen and JSFiddle also showcase user-created HTML5 games, some of which are slot machines. Additionally, specialized game development forums and communities, such as those on Reddit or Stack Overflow, often share free resources and code snippets. Always ensure to check the licensing terms to use the code legally and appropriately.
🤔 What are the best practices for designing a slot machine in HTML?
Designing a slot machine in HTML involves several best practices. First, use semantic HTML elements like